A Markplex subscriber asked what were the advantages in using methods in EasyLanguage. What is the point of them and are they really necessary? This tutorial will attempt to answer these question and gives various examples of simple methods.
Advantages of methods
Using methods in an EasyLanguage program can bring many benefits, including
1. Keeping things organized
Methods help you keep your code tidy by grouping related actions together. Imagine organizing your tools in a toolbox—methods are like the compartments that keep everything in its place, making it easier to find and use when needed.
2. Reuse your work
Once you create a method, you can use it over and over again without rewriting it. All you would need to do is copy it into the new program.
3. Breaks down complex tasks
Methods let you break down big, complicated tasks into smaller, simpler steps. It’s like assembling furniture by following separate steps for each part, making the whole process easier to manage.
4. Makes code easier to understand
Methods simplify your code by putting complex details in obvious areas of the program. This makes your code more like a well-written instruction manual—clearer and easier for others (and yourself) to follow.
5. Reduces errors
By controlling how data is used and changed, methods help prevent mistakes. It’s like having safety checks in place to make sure things don’t go wrong, keeping your program reliable.
6. Easier to fix and improve
When something needs to be fixed or updated, methods make it easier to find and change the relevant part. It’s like updating a single part of a machine without having to rebuild the whole thing.
7. Flexible and expandable
Methods allow you to build on your work easily. If you want to create new variations of your strategy, you can tweak or add methods without starting from scratch, much like adding new features to a product.
What is a method?
In EasyLanguage a method is a named subroutine within an EasyLanguage program that consists of a statement or sequence of statements to perform a calculation, plot some information or perform some other action. For example:
// Calculate the average using a simple METHOD Method Void CalcAvg( ) Begin Avg = Summation( Price, Length ) / Length; End;
is a simple method that calculates the moving average. Notice that this method returns nothing (void). What is actually does is to do a calculation and store the result in a variable. This variable was declared in the main program and its scope includes the main program and all methods contained within the main program.
The EasyLanguage statements within a method are only executed when the method is called. So the above method must be called on very bar to calculate the average on every bar, it is called using the following syntax:
// Call the CalcAvg( ) method CalcAvg( );
Scope of variables in methods
It is also possible to declare variables within methods, like in the following example:
Method double CalcAvg1( ) Vars: double Avg1; Begin Avg1 = Summation( Price, Length ) / Length; Return Avg1; End;
Note that the declaration is slightly differently from the declarations in the main program. In the method the variable datatype must be included and an initial value is not included.
This method is called using the following syntax:
Value3 = CalcAvg1( );
Where the calculated value is stored in Value3. If we try to plot Avg1 anywhere outside the method we will get an error because the scope of this variable is in the method alone.
Passing parameters to a method
In the following example a parameter (len) is passed to the method:
Method double CalcAvg2( int Len ) Vars: double Avg1; Begin Avg1 = Summation( Price, Len ) / Len; Return Avg1; End;
Best programming practice suggests that a method should reference only variables passed in through its parameter list or those declared locally within the method. However, there are instances where this approach can be cumbersome or impractical, making it acceptable to reference variables declared at the study level. So the last two methods shown will give identical results so long as Len and Length are set to the same value.
To see more examples of methods with multiple inputs and outputs see tutorial 118.
Differences between methods and functions
The main difference between EasyLanguage functions and methods is that the method is included in the same block of code as the program (i.e. indicator, show-me study, strategy, trading app etc) and is verified with the program. A function is separate piece of code that is also verified separately from the main program.
Another difference is that a method has access to variables and inputs in the program where it is contained. A function does not have access the program variables.
A function can be called by a number of different programs whereas a method is only available to the program in which it is contained. This also means that should you develop a really useful method that you want to use in another program, then you have to copy and paste the method between programs.
Use with object events
Whether you decide to use methods in your code or not, as soon as you want to use object events you will be forced to do so. For example, say we wanted to use a Price series provider and we dragged it from the toolbox to our program. If we opened its properties, clicked the event tab, and double clicked on StateChanged we would then find the following method code automatically added to our program:
method void PriceSeriesProvide1_StateChanged( elsystem.Object sender, tsdata.common.StateChangedEventArgs args ) begin end;
All event handlers have void return and two parameters. The first parameter is the “sender”, the object that caused the event and the second is the “args”, an object with event-specific properties which vary depending on the object that caused the event.
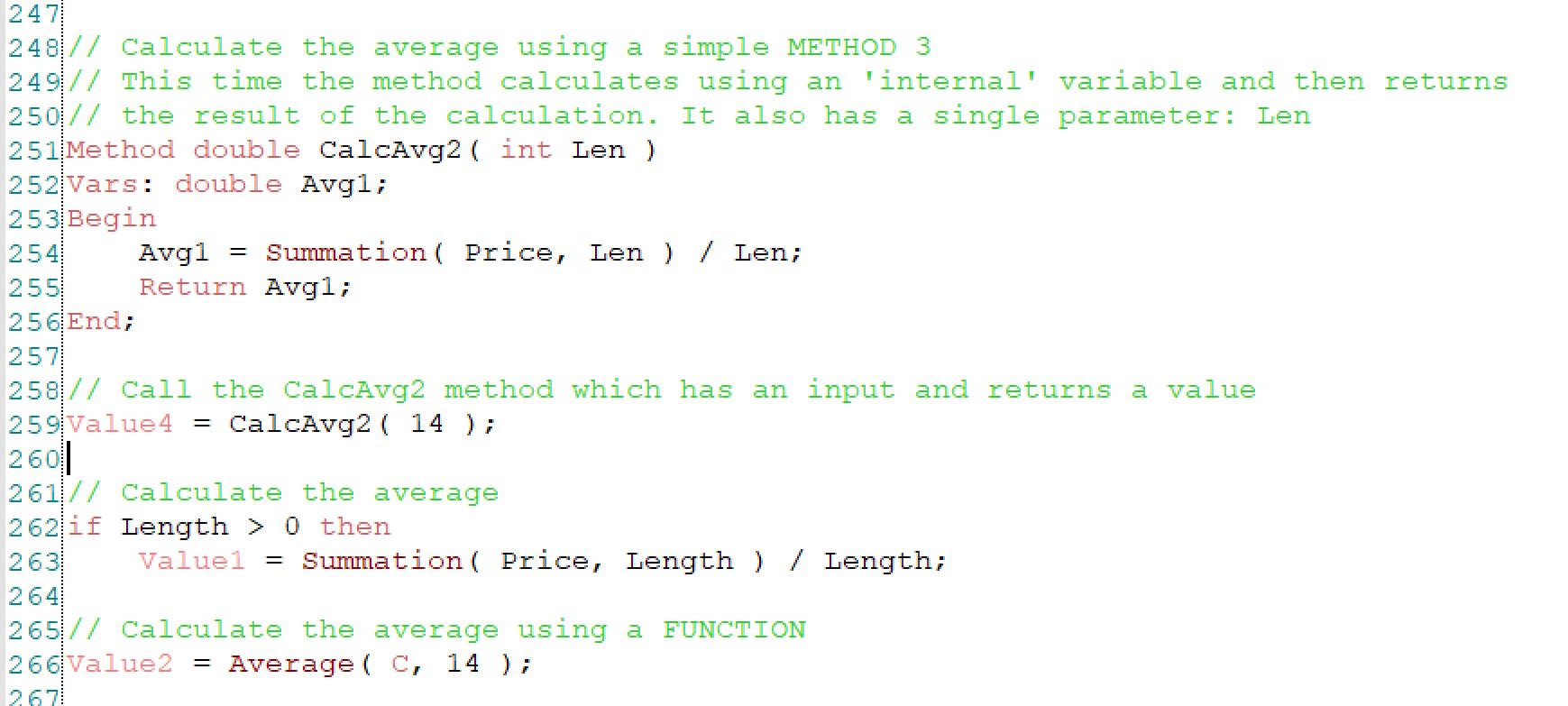
Explanation of tutorial 208
Free download of tutorial 208 example code for Gold Pass members

TO THE BEST OF MARKPLEX CORPORATION’S KNOWLEDGE, ALL OF THE INFORMATION ON THIS PAGE IS CORRECT, AND IT IS PROVIDED IN THE HOPE THAT IT WILL BE USEFUL. HOWEVER, MARKPLEX CORPORATION ASSUMES NO LIABILITY FOR ANY DAMAGES, DIRECT OR OTHERWISE, RESULTING FROM THE USE OF THIS INFORMATION AND/OR PROGRAM(S) DESCRIBED, AND NO WARRANTY IS MADE REGARDING ITS ACCURACY OR COMPLETENESS. USE OF THIS INFORMATION AND/OR PROGRAMS DESCRIBED IS AT YOUR OWN RISK.
ANY EASYLANGUAGE OR POWERLANGUAGE TRADING STRATEGIES, SIGNALS, STUDIES, INDICATORS, SHOWME STUDIES, PAINTBAR STUDIES, PROBABILITYMAP STUDIES, ACTIVITYBAR STUDIES, FUNCTIONS (AND PARTS THEREOF) AND ASSOCIATED TECHNIQUES REFERRED TO, INCLUDED IN OR ATTACHED TO THIS TUTORIAL OR PROGRAM DESCRIPTION ARE EXAMPLES ONLY, AND HAVE BEEN INCLUDED SOLELY FOR EDUCATIONAL PURPOSES. MARKPLEX CORPORATION. DOES NOT RECOMMEND THAT YOU USE ANY SUCH TRADING STRATEGIES, SIGNALS, STUDIES, INDICATORS, SHOWME STUDIES, PAINTBAR STUDIES, PROBABILITYMAP STUDIES, ACTIVITYBAR STUDIES, FUNCTIONS (OR ANY PARTS THEREOF) OR TECHNIQUES. THE USE OF ANY SUCH TRADING STRATEGIES, SIGNALS, STUDIES, INDICATORS, SHOWME STUDIES, PAINTBAR STUDIES, PROBABILITYMAP STUDIES, ACTIVITYBAR STUDIES, FUNCTIONS AND TECHNIQUES DOES NOT GUARANTEE THAT YOU WILL MAKE PROFITS, INCREASE PROFITS, OR MINIMIZE LOSSES.